content
stringlengths 35
416k
| sha1
stringlengths 40
40
| id
int64 0
710k
|
---|---|---|
def getTopApSignals(slot_to_io):
""" HLS simulator requires that there is an ap_done at the top level """
# find which slot has the s_axi_control
for slot, io_list in slot_to_io.items():
if any('s_axi' in io[-1] for io in io_list):
# note the naming convention
ap_done_source = [f'{io[-1]}_in' for io in io_list if 'ap_done' in io[-1]]
ap_start_source = [f'{io[-1]}_out' for io in io_list if 'ap_start' in io[-1]]
top_ap_signals = []
top_ap_signals.append(f'wire ap_done = ' + ' & '.join(ap_done_source) + ';')
top_ap_signals.append('wire ap_idle = ap_done;')
top_ap_signals.append('wire ap_ready = ap_done;')
top_ap_signals.append(f'wire ap_start = {ap_start_source[0]};') # only need 1 ap_start
return top_ap_signals
assert False | e40a8fb7797653ee7414c0120ceb29e49e9dfd84 | 3,380 |
from typing import Dict
from pathlib import Path
import inspect
import json
def load_schema(rel_path: str) -> Dict:
"""
Loads a schema from a relative path of the caller of this function.
:param rel_path: Relative path from the caller. e.g. ../schemas/schema.json
:return: Loaded schema as a `dict`.
"""
caller_path = Path((inspect.stack()[1])[1]).parent
fp = (caller_path / rel_path).resolve()
with open(fp, "r") as fh:
data = json.loads(fh.read())
return data | 297e0e01dd2f4af071ab99ebaf203ddb64525c89 | 3,381 |
def hsla_to_rgba(h, s, l, a):
""" 0 <= H < 360, 0 <= s,l,a < 1
"""
h = h % 360
s = max(0, min(1, s))
l = max(0, min(1, l))
a = max(0, min(1, a))
c = (1 - abs(2*l - 1)) * s
x = c * (1 - abs(h/60%2 - 1))
m = l - c/2
if h<60:
r, g, b = c, x, 0
elif h<120:
r, g, b = x, c, 0
elif h<180:
r, g, b = 0, c, x
elif h<240:
r, g, b = 0, x, c
elif h<300:
r, g, b = x, 0, c
else:
r, g, b = c, 0, x
return (int((r+m)*255), int((g+m)*255), int((b+m)*255), int(a*255)) | 55e546756d4dd2a49581a5f950beb286dd73f3f9 | 3,383 |
from typing import Dict
from pathlib import Path
from typing import Optional
def prioritize(paths: Dict[int, Path], purpose: str) -> Optional[Path]:
"""Returns highest-priority and existing filepath from ``paths``.
Finds existing configuration or data file in ``paths`` with highest
priority and returns it, otherwise returns ``None``.
"""
for key in sorted(paths.keys(), reverse=True):
if purpose == "config":
if paths[key].exists():
return paths[key]
if purpose == "data":
return paths[key] | 2c00d0bfe696040c2c19dc1d8b3393b7be124e11 | 3,384 |
def traverse(d, path):
"""Return the value at the given path from the given nested dict/list"""
for k in path.split('.'):
if k.isdigit():
k = int(k)
d = d[k]
return d | ba832a008073da5d97ba0a237a8e0ded17e4694e | 3,385 |
def reflect(array, holder=1):
"""
Reflects a np array across the y-axis
Args:
array: array to be reflected
holder: a holder variable so the function can be used in optimization algorithms. If <0.5, does not reflect.
Returns:
Reflected array
"""
c = array.copy()
if holder > 0.5:
c[:, 0] = -c[:, 0]
return c | c39cbf0bb3a949254e4f0c35b20bdf84766d2084 | 3,387 |
from typing import List
def is_common_prefix(words: List[str], length: int) -> bool:
"""Binary Search"""
word: str = words[0][:length]
for next_word in words[1:]:
if not next_word.startswith(word):
return False
return True | f57c7309f725baba0b65c92181a6f1ab2827558a | 3,389 |
def mode_mods_to_int(mode: str) -> int:
"""Converts mode_mods (str) to mode_mods (int)."""
# NOTE: This is a temporary function to convert the leaderboard mode to an int.
# It will be removed when the site is fully converted to use the new
# stats table.
for mode_num, mode_str in enumerate((
'vn_std', 'vn_taiko', 'vn_catch', 'vn_mania',
'rx_std', 'rx_taiko', 'rx_catch',
'ap_std'
)):
if mode == mode_str:
return mode_num
else:
return 0 | 0bfaa8cf04bcee9395dff719067be9753be075c4 | 3,390 |
def get_path_to_spix(
name: str,
data_directory: str,
thermal: bool,
error: bool = False,
file_ending: str = "_6as.fits",
) -> str:
"""Get the path to the spectral index
Args:
name (str): Name of the galaxy
data_directory (str): dr2 data directory
thermal (bool): non thermal data
error (bool): path to error
file_ending (str, optional): File ending. Defaults to ".fits".
Returns:
str: [description]
"""
return f"{data_directory}/magnetic/{name}/{name}_spix{'_non_thermal' if thermal else ''}{'_error' if error else ''}{file_ending}" | bf8fdff001049ed0738ed856e8234c43ce4511b7 | 3,391 |
from bs4 import BeautifulSoup
def parse_object_properties(html):
"""
Extract key-value pairs from the HTML markup.
"""
if isinstance(html, bytes):
html = html.decode('utf-8')
page = BeautifulSoup(html, "html5lib")
propery_ps = page.find_all('p', {'class': "list-group-item-text"})
obj_props_dict = {}
for p in propery_ps:
if 'data-name' in p.attrs:
key = p.attrs['data-name']
value = p.get_text().strip()
obj_props_dict[key] = value
return obj_props_dict | 8eb2d15cb5f46075ec44ff61265a8f70123a8646 | 3,392 |
def model_choices_from_protobuf_enum(protobuf_enum):
"""Protobufs Enum "items" is the opposite order djagno requires"""
return [(x[1], x[0]) for x in protobuf_enum.items()] | d3f5431293a9ab3fdf9a92794b1225a0beec40cc | 3,393 |
from bs4 import BeautifulSoup
def parseHtml(html):
"""
BeautifulSoup でパースする
Parameters
----------
html : str
HTML ソース文字列
Returns
-------
soup : BeautifulSoup
BeautifulSoup オブジェクト
"""
soup = BeautifulSoup(html, 'html.parser')
return soup | e8d7a39a9881606d1dfee810ab1c2cecd11eaba2 | 3,395 |
def generate_modal(title, callback_id, blocks):
"""
Generate a modal view object using Slack's BlockKit
:param title: Title to display at the top of the modal view
:param callback_id: Identifier used to help determine the type of modal view in future responses
:param blocks: Blocks to add to the modal view
:return: View object (Dictionary)
"""
modal = {
"type": "modal",
"callback_id": callback_id,
"title": {
"type": "plain_text",
"text": title,
"emoji": False
},
"submit": {
"type": "plain_text",
"text": "Submit",
"emoji": False
},
"close": {
"type": "plain_text",
"text": "Cancel",
"emoji": False
},
"blocks": blocks
}
return modal | e0caeec1ab1cf82ed6f02ec77a984dcb25e329f5 | 3,399 |
import os
def bq_use_legacy_sql():
"""
Returns BIGQUERY_LEGACY_SQL if env is set
"""
return os.environ.get('BIGQUERY_LEGACY_SQL', 'TRUE') | 53d51c5eb1caa9b6041e9b2d29bde068199bba52 | 3,400 |
def linkCount(tupleOfLists, listNumber, lowerBound, upperBound):
"""Counts the number of links in one of the lists passed.
This function is a speciality function to aid in calculating
statistics involving the number of links that lie in a given
range. It is primarily intended as a private helper function. The
parameters are:
tupleOfLists -- usually a linkograph entry.
listNumber -- a list of the indicies in entry that should be
considered.
lowerBound -- the lowest index that should be considered.
upperBound -- the highest index that should be considered.
Example: a typical tupleOfLists is ({'A', 'B'}, {1,2}, {4,5}) a
listNumber of [1] would only consider the links in {1,2}, a
listNumber of [2] would only consider the links in {4,5} and a
listNumber of [1,2] would consider the links in both {1,2}, and
{4,5}.
"""
summation = 0
for index in listNumber:
summation += len({link for link in tupleOfLists[index]
if link >= lowerBound
and link <= upperBound})
return summation | 239fd8d3c01fe6c88444cfa7369459e3c76005dc | 3,401 |
def computeLPS(s, n):
"""
Sol with better comle
"""
prev = 0 # length of the previous longest prefix suffix
lps = [0]*(n)
i = 1
# the loop calculates lps[i] for i = 1 to n-1
while i < n:
if s[i] == s[prev]:
prev += 1
lps[i] = prev
i += 1
else:
# This is tricky. Consider the example.
# AAACAAAA and i = 7. The idea is similar
# to search step.
if prev != 0:
prev = lps[prev-1]
# Also, note that we do not increment i here
else:
lps[i] = 0
i += 1
print(lps)
return lps[n-1] | 8b4374c9ac29f59cf1f4b0e6e07628776828c11a | 3,402 |
import argparse
def parse_args():
"""
Argument Parser
"""
parser = argparse.ArgumentParser(description="Wiki Text Extractor")
parser.add_argument("-i", "--input_dir", dest="input_dir", type=str, metavar="PATH",
default="./extracted",help="Input directory path ")
parser.add_argument("-o", "--output_dir", dest="output_dir", type=str, metavar="PATH",
default="./wiki_text",help="Output directory path")
parser.add_argument("-t", "--output_type", dest="output_type", type=int, metavar="INT",
default=1, choices=[1,2], help="Output in a single file or multiple file")
args = parser.parse_args()
return args | af9149f893bb652c7e86a731749b3e2a5e4c1c8f | 3,403 |
import textwrap
import sys
def wrap_text(translations, linewrap=0):
"""Pretty print translations.
If linewrap is set to 0 disble line wrapping.
Parameters
----------
translations : list
List of word translations.
linewrap : int
Maximum line length before wrapping.
"""
# pylint: disable=too-many-locals
def wrap(text, width=linewrap, findent=0, sindent=0, bold=False):
if width == 0:
text = " " * findent + text
else:
text = textwrap.fill(
text,
width=width,
initial_indent=" " * findent,
subsequent_indent=" " * sindent,
)
# don't use bold when stdout is pipe or redirect
if bold and sys.stdout.isatty():
text = "\033[0;1m" + text + "\033[0m"
return text
indent = 5
result = []
for i1, trans in enumerate(translations):
if i1 > 0:
result.append("\n")
for w in trans.word:
result.append(wrap(w, bold=True))
for i2, t in enumerate(trans.parts_of_speech):
if i2 > 0:
result.append("")
if t.part:
result.append("[{part}]".format(part=t.part))
for i3, m in enumerate(t.meanings, 1):
if i3 > 1:
result.append("")
meaning = "{index:>3}. {meanings}".format(
index=i3, meanings=", ".join(m.meaning)
)
result.append(wrap(meaning, sindent=indent, bold=True))
eindent = indent + 1
for e in m.examples:
result.append("")
result.append(wrap(e[0], findent=eindent, sindent=eindent))
if len(e) == 2 and e[1]:
result.append(wrap(e[1], findent=eindent, sindent=eindent + 1))
return "\n".join(result) | adecfba77f017d4888fffda43c0466e9125b43ea | 3,405 |
import logging
import os
import subprocess
def run(
command,
cwd=None,
capture_output=False,
input=None,
check=True,
**subprocess_run_kwargs
):
""" Wrapper for subprocess.run() that sets some sane defaults """
logging.info("Running {} in {}".format(" ".join(command), cwd or os.getcwd()))
if isinstance(input, str):
input = input.encode("utf-8")
env = os.environ.copy()
env["HOMEBREW_NO_AUTO_UPDATE"] = "1"
return subprocess.run(
command,
cwd=cwd,
input=input,
stdout=subprocess.PIPE if capture_output else None,
check=check,
env=env,
**subprocess_run_kwargs
) | 5595721fc28da8a87230a1d367cbc5c3bacdc779 | 3,406 |
def construct_gpu_info(statuses):
""" util for unit test case """
m = {}
for status in statuses:
m[status.minor] = status
m[status.uuid] = status
return m | b8b2f41799b863d2e22066005b901f17a610d858 | 3,407 |
def my_vtk_grid_props(vtk_reader):
"""
Get grid properties from vtk_reader instance.
Parameters
----------
vtk_reader: vtk Reader instance
vtk Reader containing information about a vtk-file.
Returns
----------
step_x : float
For regular grid, stepsize in x-direction.
step_y : float
For regular grid, stepsize in y-direction.
npts_x : float
Number of cells in x-direction.
npts_y : float
Number of cells in y-direction.
low_m_x : float
Middle of first x cell
high_m_x : float
Middle of last x cell
low_m_y : float
Middle of first y cell
high_m_y : float
Middle of last y cell
low_x : float
Edge of first x cell
high_x : float
Edge of last x cell
low_y : float
Edge of first y cell
high_y : float
Edge of last y cell
Notes
----------
0: step_x
1: step_y
2: npts_x
3: npts_y
4: low_m_x - Middle of cells: first x cell
5: high_m_x - Middle of cells: last x cell
6: low_m_y - Middle of cells: first y cell
7: high_m_y - Middle of cells: last y cell
8: low_x - Edge of cells: first x cell
9: high_x - Edge of cells: last x cell
10: low_y - Edge of cells: first y cell
11: high_y - Edge of cells: last y cell
"""
vtk_output = vtk_reader.GetOutput()
# Read attributes of the vtk-Array
# num_cells = vtk_output.GetNumberOfCells()
# num_points = vtk_output.GetNumberOfPoints()
# whole_extent = vtk_output.GetExtent()
grid_bounds = vtk_output.GetBounds()
grid_dims = vtk_output.GetDimensions()
# Grid information
step_x = (grid_bounds[1] - grid_bounds[0]) / (grid_dims[0] - 1)
step_y = (grid_bounds[3] - grid_bounds[2]) / (grid_dims[1] - 1)
if grid_bounds[0] == 0.0: # CELLS
npts_x = grid_dims[0] - 1
npts_y = grid_dims[1] - 1
low_m_x = grid_bounds[0] + 0.5 * step_x
high_m_x = grid_bounds[1] - 0.5 * step_x
low_m_y = grid_bounds[2] + 0.5 * step_y
high_m_y = grid_bounds[3] - 0.5 * step_y
low_x = grid_bounds[0]
high_x = grid_bounds[1]
low_y = grid_bounds[2]
high_y = grid_bounds[3]
else: # POINTS
npts_x = grid_dims[0]
npts_y = grid_dims[1]
low_m_x = grid_bounds[0]
high_m_x = grid_bounds[1]
low_m_y = grid_bounds[2]
high_m_y = grid_bounds[3]
low_x = grid_bounds[0] - 0.5 * step_x
high_x = grid_bounds[1] + 0.5 * step_x
low_y = grid_bounds[2] - 0.5 * step_y
high_y = grid_bounds[3] + 0.5 * step_y
return step_x, step_y, \
npts_x, npts_y, \
low_m_x, high_m_x, low_m_y, high_m_y, \
low_x, high_x, low_y, high_y | 26ef8a51648ea487372ae06b54c8ccf953aeb414 | 3,408 |
def state_space_model(A, z_t_minus_1, B, u_t_minus_1):
"""
Calculates the state at time t given the state at time t-1 and
the control inputs applied at time t-1
"""
state_estimate_t = (A @ z_t_minus_1) + (B @ u_t_minus_1)
return state_estimate_t | 0e04207028df8d4162c88aad6606e792ef618f5a | 3,409 |
from typing import Callable
import re
def check_for_NAs(func: Callable) -> Callable:
"""
This decorator function checks whether the input string qualifies as an
NA. If it does it will return True immediately. Otherwise it will run
the function it decorates.
"""
def inner(string: str, *args, **kwargs) -> bool:
if re.fullmatch("^|0|NA$", string) is not None:
return True
else:
return func(string, *args, **kwargs)
return inner | e9336cca2e6cd69f81f6aef1d11dc259492774f8 | 3,410 |
import random
def _get_name(filename: str) -> str:
"""
Function returns a random name (first or last)
from the filename given as the argument.
Internal function. Not to be imported.
"""
LINE_WIDTH: int = 20 + 1 # 1 for \n
with open(filename) as names:
try:
total_names = int(next(names))
nth_name_to_read: int = random.randint(1, total_names)
# Here 'nth_name_to_read' lines are skipped that include
# the first line (with no of lines) and n-1 names
# Next read would always be the desired name
bytes_to_seek: int = LINE_WIDTH * nth_name_to_read
_ = names.seek(bytes_to_seek) # Now skipped n - 1 names
name: str = next(names).strip()
return name
except StopIteration:
# Return empty string if the file is empty
return '' | 1b4cd75488c6bd1814340aee5669d1631318e77f | 3,411 |
def get_n_runs(slurm_array_file):
"""Reads the run.sh file to figure out how many conformers or rotors were meant to run
"""
with open(slurm_array_file, 'r') as f:
for line in f:
if 'SBATCH --array=' in line:
token = line.split('-')[-1]
n_runs = 1 + int(token.split('%')[0])
return n_runs
return 0 | 5574ef40ef87c9ec5d9bbf2abd7d80b62cead2ab | 3,412 |
def calc_field_changes(element, np_id):
"""
Walk up the tree of geo-locations, finding the new parents
These will be set onto all the museumobjects.
"""
fieldname = element._meta.concrete_model.museumobject_set.\
related.field.name
field_changes = {}
field_changes[fieldname] = element.id
if hasattr(element, 'parent'):
field_changes.update(
calc_field_changes(element.parent, element.parent.id))
return field_changes | cba816488dcf10a774bc18b1b3f6498e1d8dc3d8 | 3,414 |
def __setAdjacent_square__(self, pos):
"""
Sets all adjacencies in the map for a map with square tiles.
"""
self.__checkIndices__(pos)
i, j = pos; adjacent = []
# Function to filter out nonexistent cells.
def filterfn(p):
do_not_filter = 0 <= p[0] < self.__numrows__ and 0 <= p[1] < self.__numcols__
return do_not_filter and not self.__isdisabled__[p[0]][p[1]]
for cell in filter(filterfn, ( (i+1,j), (i-1,j), (i,j+1), (i,j-1) )):
adjacent += [cell]
self.__adjacent__[i][j] = adjacent | ebdd3ee3d0104b5bd26cc48e07760de027615263 | 3,417 |
def rule_if_system(system_rule, non_system_rule, context):
"""Helper function to pick a rule based on system-ness of context.
This can be used (with functools.partial) to choose between two
rule names, based on whether or not the context has system
scope. Specifically if we will fail the parent of a nested policy
check based on scope_types=['project'], this can be used to choose
the parent rule name for the error message check in
common_policy_check().
"""
if context.system_scope:
return system_rule
else:
return non_system_rule | 2149c2ffdd6afdd64f7d33a2de4c6a23b3143dee | 3,418 |
def returns_unknown():
"""Tuples are a not-supported type."""
return 1, 2, 3 | 9fc003c890b4e053362c684b1a5f0dfca59bbe42 | 3,420 |
def _make_hours(store_hours):
"""Store hours is a dictionary that maps a DOW to different open/close times
Since it's easy to represent disjoing hours, we'll do this by default
Such as, if a store is open from 11am-2pm and then 5pm-10pm
We'll slice the times in to a list of floats representing 30 minute intevals
So for monday, let's assume we have the store hours from 10am - 3pm
We represent this as
monday = [10.0, 10.5, 11.0, 11.5, 12.0, 12.5, 13.0, 13.5, 14.0, 14.5]
"""
week_hrs = {}
for dow in store_hours.keys():
dow_hours = []
for hour_set in store_hours[dow]:
if len(hour_set) < 2:
open_hr = 0.0
close_hr = 24.0
else:
open_hr = float(hour_set[0])
close_hr = float(hour_set[1])
if close_hr < open_hr:
tmp = close_hr
close_hr = open_hr
open_hr = tmp
current_hr_it = open_hr
while((close_hr - current_hr_it) >= .5):
dow_hours.append(current_hr_it)
current_hr_it += .5
week_hrs[dow] = dow_hours
return week_hrs | 4845594e59e5dba2790ac1a3c376ddb8e8290995 | 3,421 |
def update_image_version(name: str, new_version: str):
"""returns the passed image name modified with the specified version"""
parts = name.rsplit(':', 1)
return f'{parts[0]}:{new_version}' | cde798361a6c74d22f979fe013e963c46028a7e6 | 3,425 |
def dir_name(dir_path):
"""
转换零时文件夹、输入文件夹路径
:param dir_path: 主目录路径
:return:[tmp_dir, input_dir, res_dir]
"""
tmp_dir = dir_path + "tmp\\"
input_dir = dir_path + "input\\"
res_dir = dir_path + "result\\"
return tmp_dir, input_dir, res_dir | 9f775b4ace14b178fd7bc0dfa94e5df13e583557 | 3,426 |
def _gen_roi_func_constant(constant_roi):
"""
Return a RoI function which returns a constant radius.
See :py:func:`map_to_grid` for a description of the parameters.
"""
def roi(zg, yg, xg):
""" constant radius of influence function. """
return constant_roi
return roi | c7c69cf32fb289d5e9c9497474989aa873a231ba | 3,427 |
import torch
def absolute_filter_change(baseline_state_dict, target_state_dict):
""" Calculate sum(abs(K2 - K1) / sum(K1))
Args:
baseline_state_dict (dict): state_dict of ori_net
target_state_dict (dict): state_dict of finetune_net
Returns:
sorted_diff (list): sorted values
sorted_index (list): sorted index of kernel
"""
# save all weight to list
baseline_weight_list = []
for key, value in baseline_state_dict.items():
if key.find('weight') != -1:
weight = value.reshape(-1, 3, 3)
baseline_weight_list.append(weight)
# [-1, 3, 3]
baseline_weight_list = torch.cat(baseline_weight_list, dim=0)
target_weight_list = []
for key, value in target_state_dict.items():
if key.find('weight') != -1:
weight = value.reshape(-1, 3, 3)
target_weight_list.append(weight)
# [-1, 3, 3]
target_weight_list = torch.cat(target_weight_list, dim=0)
sum_baseline_weight = torch.sum(torch.sum(abs(baseline_weight_list), dim=1), dim=1)
sum_baseline_weight = sum_baseline_weight.unsqueeze(1).unsqueeze(1)
diff = torch.sum(torch.sum(abs(target_weight_list - baseline_weight_list) / sum_baseline_weight, dim=1), dim=1)
return diff.cpu().numpy() | ad4616a03ef80f5a5430a87fd07d70d6bb10f7b7 | 3,429 |
import torch
def load_checkpoints(checkpoint_name):
"""
Load a pretrained checkpoint.
:param checkpoint_name: checkpoint filename
:return: model.state_dict, source_vocabulary, target_vocabulary,
"""
# Get checkpoint from file
checkpoint = torch.load(checkpoint_name, map_location=torch.device('cpu'))
# The epoch when training has been left
epoch = checkpoint['epoch']
# The time elapsed during training
time_elapsed = checkpoint['time_elapsed']
# Get state_dict of the model
model_state_dict = checkpoint['model_state_dict']
# Get the state_dict of the optimizer
optimizer_state_dict = checkpoint['optimizer_state_dict']
# Get source language vocabulary
src_vocabulary = checkpoint['src_vocabulary']
tgt_vocabulary = checkpoint['tgt_vocabulary']
return model_state_dict, optimizer_state_dict, epoch, time_elapsed, src_vocabulary, tgt_vocabulary | e81f094c811d497504fd1f93a8ee537e6b122bd6 | 3,430 |
def _extract_data(prices, n_markets):
""" Extract the open, close, high and low prices from the price matrix. """
os = prices[:, :, :n_markets]
cs = prices[:, :, n_markets:2*n_markets]
hs = prices[:, :, 2*n_markets:3*n_markets]
ls = prices[:, :, 3*n_markets:4*n_markets]
return os, cs, hs, ls | 154af0c8270fbe664b3dd5d07a724b753ff02040 | 3,431 |
import os
def listFiles(sDir,ext="_du.mpxml"):
"""
return 1 list of files
"""
lsFile = sorted([_fn
for _fn in os.listdir(sDir)
if _fn.lower().endswith(ext) or _fn.lower().endswith(ext)
])
return lsFile | 873f331db44a96fe9d826dbc926354ce4ded6542 | 3,432 |
import base64
import hmac
import hashlib
def sign_v2(key, msg):
"""
AWS version 2 signing by sha1 hashing and base64 encode.
"""
return base64.b64encode(hmac.new(key, msg.encode("utf-8"), hashlib.sha1).digest()) | 1aa54cc2cd3ce20ad5222a889754efda2f4632c3 | 3,435 |
def find_hcf(a, b) :
""" Finds the Highest Common Factor among two numbers """
#print('HCF : ', a, b)
if b == 0 :
return a
return find_hcf(b, a%b) | 818bbc05ab9262e8fd1e8975daf68ca3e0fa6a8b | 3,436 |
def version_compare(a, b): # real signature unknown; restored from __doc__
"""
version_compare(a: str, b: str) -> int
Compare the given versions; return a strictly negative value if 'a' is
smaller than 'b', 0 if they are equal, and a strictly positive value if
'a' is larger than 'b'.
"""
return 0 | 97b3fd3bbd542d776b75327c88f9e80d776ba248 | 3,437 |
def query_title_bar_text(shared_state):
"""return text for title bar, updated when screen changes."""
coll_name = shared_state["active_collection"].name
str_value = f"QUERY SOURCE: {coll_name}"
return str_value | 2ce051cc8d6a87d3c964fba1abb502125b227717 | 3,440 |
def GetInput():
"""Get player inputs and lower-case the input"""
Input = str(input("{:>20s}".format("")))
print("\n \n \n \n \n")
return Input.lower() | 9d8626a9c9f0615a0453d0804b6b37244ec373c3 | 3,441 |
def stripper(reply: str, prefix=None, suffix=None) -> str:
"""This is a helper function used to strip off reply prefix and
terminator. Standard Python str.strip() doesn't work reliably because
it operates on character-by-character basis, while prefix/terminator
is usually a group of characters.
Args:
reply: String to be stripped.
prefix: Substring to remove from the beginning of the line.
suffix: Substring to remove from the end of the line.
Returns:
(str): Naked reply.
"""
if prefix is not None and reply.startswith(prefix):
reply = reply[len(prefix):]
if suffix is not None and reply.endswith(suffix):
reply = reply[:-len(suffix)]
return reply | b48281a0dedd5d7f3d476943f12ac49720e67476 | 3,442 |
import re
def trimBody(body):
""" Quick function for trimming away the fat from emails """
# Cut away "On $date, jane doe wrote: " kind of texts
body = re.sub(
r"(((?:\r?\n|^)((on .+ wrote:[\r\n]+)|(sent from my .+)|(>+[ \t]*[^\r\n]*\r?\n[^\n]*\n*)+)+)+)",
"",
body,
flags=re.I | re.M,
)
# Crop out quotes
lines = body.split("\n")
body = "\n".join([x for x in lines if not x.startswith(">")])
# Remove hyperlinks
body = re.sub(r"[a-z]+://\S+", "", body)
# Remove email addresses
body = re.sub(r"(<[^>]+>\s*\S+@\S+)", "", body)
body = re.sub(r"(\S+@\S+)", "", body)
return body | 19fcb7313e66d7e710781cf195a7550d050b4848 | 3,443 |
import os
def custom_client_datalist_json_path(datalist_json_path: str, client_id: str, prefix: str) -> str:
"""
Customize datalist_json_path for each client
Args:
datalist_json_path: default datalist_json_path
client_id: e.g., site-2
"""
# Customize datalist_json_path for each client
# - client_id: e.g. site-5
head, tail = os.path.split(datalist_json_path)
datalist_json_path = os.path.join(
head,
prefix + "_" + str(client_id) + ".json",
)
return datalist_json_path | fb21864a18e105bbfe73cbec75ebcfd4ac52910b | 3,444 |
def advertisement_data_complete_builder(list_of_ad_entries):
"""
Generate a finalized advertisement data value from a list of AD entries that can be passed
to the BLEConnectionManager to set the advertisement data that is sent during advertising.
:param list_of_ad_entries: List of AD entries (can be built using blesuite.utils.gap_utils.advertisement_data_entry_builder)
:type list_of_ad_entries: [str,]
:return: Finalized AD data
:rtype: str
"""
data = ""
for ad in list_of_ad_entries:
length = len(ad)
ad_string = chr(length) + ad
data = data + ad_string
return data | c0f9040c36216cb519706c347d6644405fae0b7f | 3,445 |
import math
def quantize(x):
"""convert a float in [0,1] to an int in [0,255]"""
y = math.floor(x*255)
return y if y<256 else 255 | b941a11d0d6af3162c964568e2d97c8d81cd1442 | 3,446 |
def result(a, b, operator):
"""This function return result"""
lambda_ops = {
"+": (lambda x,y: x+y),
"-": (lambda x,y: x-y),
"*": (lambda x,y: x*y),
"/": (lambda x,y: x/y),
"//": (lambda x,y: x//y),
"%": (lambda x,y: x%y),
}
r = False
error = ''
if operator in lambda_ops:
if (operator == "/" or operator == "//" or operator == "%" ) and b==0:
error = "Oops, division or modulo by zero"
else:
r = lambda_ops[operator](a, b)
else:
error = "Use either + - * / or % next time"
return r, error | febfacf3aa94bc15931cf79979329b3b1a5c7bc5 | 3,447 |
def points(piece_list):
"""Calculating point differential for the given board state"""
# Args: (1) piece list
# Returns: differential (white points - black points)
# The points are calculated via the standard chess value system:
# Pawn = 1, King = 3, Bishop = 3, Rook = 5, Queen = 9
# King = 100 (arbitrarily large)
differential = 0
# For all white pieces...
for i in range(0,16):
# If the piece is active, add its points to the counter
if piece_list[i].is_active:
differential = differential + piece_list[i].value
# For all black pieces...
for i in range(16,32):
# If the piece is active, subtract its points from the counter
if piece_list[i].is_active:
differential = differential - piece_list[i].value
# Return point differential
return differential | d8f36fd887a846a20999a0a99ad672d2902473d4 | 3,448 |
def get_package_version() -> str:
"""Returns the package version."""
metadata = importlib_metadata.metadata(PACKAGE_NAME) # type: ignore
version = metadata["Version"]
return version | a24286ef2a69f60871b41eda8e5ab39ba7f756c0 | 3,449 |
def safe_name(dbname):
"""Returns a database name with non letter, digit, _ characters removed."""
char_list = [c for c in dbname if c.isalnum() or c == '_']
return "".join(char_list) | 2ce4978c3467abaddf48c1d1ab56ed773b335652 | 3,450 |
def concatenate_shifts(shifts):
""" Take the shifts, which are relative to the previous shift,
and sum them up so that all of them are relative to the first."""
# the first shift is 0,0,0
for i in range(2, len(shifts)): # we start at the third
s0 = shifts[i-1]
s1 = shifts[i]
s1.x += s0.x
s1.y += s0.y
s1.z += s0.z
return shifts | f4b0a41db1db78e3b5f25ca198fdb6cebd6476ca | 3,451 |
from typing import Any
def next_key(basekey: str, keys: dict[str, Any]) -> str:
"""Returns the next unused key for basekey in the supplied dictionary.
The first try is `basekey`, followed by `basekey-2`, `basekey-3`, etc
until a free one is found.
"""
if basekey not in keys:
return basekey
i = 2
while f"{basekey}-{i}" in keys:
i = i + 1
return f"{basekey}-{i}" | e1da51c79fd465088294e053fdc970934268211b | 3,452 |
import yaml
def load_mmio_overhead_elimination_map(yaml_path):
"""
Load a previously dumped mmio overhead elimination map
"""
with open(yaml_path, "r") as yaml_file:
res = yaml.safe_load(yaml_file.read())
res_map = {
'overall': res[0]['overall'],
'per_model': res[1]['per_model'],
}
if len(res) > 2:
res_map['per_access_context'] = res[2]['per_access_context']
return res_map | ae0ead1aa8c9f26acad9a23a35791592efbfe47e | 3,453 |
def join_b2_path(b2_dir, b2_name):
"""
Like os.path.join, but for B2 file names where the root directory is called ''.
:param b2_dir: a directory path
:type b2_dir: str
:param b2_name: a file name
:type b2_name: str
"""
if b2_dir == '':
return b2_name
else:
return b2_dir + '/' + b2_name | 20f4e6e54f7f3b4a1583b503d4aa2d8995318978 | 3,454 |
def mjd2crnum(mjd):
"""
Converts MJD to Carrington Rotation number
Mathew Owens, 16/10/20
"""
return 1750 + ((mjd-45871.41)/27.2753) | 233f91e6de4c5105732fc2c8f9f33d054491e1d2 | 3,456 |
def poly_print_simple(poly,pretty=False):
"""Show the polynomial in descending form as it would be written"""
# Get the degree of the polynomial in case it is in non-normal form
d = poly.degree()
if d == -1:
return f"0"
out = ""
# Step through the ascending list of coefficients backward
# We do this because polynomials are usually written in descending order
for pwr in range(d,-1,-1):
# Skip the zero coefficients entirely
if poly[pwr] == 0:
continue
coe = poly[pwr]
val = abs(coe)
sgn = "-" if coe//val == -1 else "+"
# When the coefficient is 1 or -1 don't print it unless it is the
# coefficient for x^0
if val == 1 and pwr != 0:
val = ""
# If it is the first term include the sign of the coefficient
if pwr == d:
if sgn == "+":
sgn = ""
# Handle powers of 1 or 0 that appear as the first term
if pwr == 1:
s = f"{sgn}{val}x"
elif pwr == 0:
s = f"{sgn}{val}"
else:
if pretty == False:
s = f"{sgn}{val}x^{pwr}"
else:
s = f"{sgn}{val}x$^{{{pwr}}}$"
# If the power is 1 just show x rather than x^1
elif pwr == 1:
s = f" {sgn} {val}x"
# If the power is 0 only show the sign and value
elif pwr == 0:
s = f" {sgn} {val}"
# Otherwise show everything
else:
if pretty == False:
s = f" {sgn} {val}x^{pwr}"
else:
s = f" {sgn} {val}x$^{{{pwr}}}$"
out += s
return out | 903f9d4a703e3f625da5f13f6fe084e8894d723b | 3,457 |
def newNXentry(parent, name):
"""Create new NXentry group.
Args:
parent (h5py.File or h5py.Group): hdf5 file handle or group
group (str): group name without extension (str)
Returns:
hdf5.Group: new NXentry group
"""
grp = parent.create_group(name)
grp.attrs["NX_class"] = "NXentry"
if "NX_class" in parent.attrs:
if parent.attrs["NX_class"] == "NXentry":
grp.attrs["NX_class"] = "NXsubentry"
return grp | 1529fbe80ca8a23f8cd7717c5df5d7d239840149 | 3,458 |
import torch
def vnorm(velocity, window_size):
"""
Normalize velocity with latest window data.
- Note that std is not divided. Only subtract mean
- data should have dimension 3.
"""
v = velocity
N = v.shape[1]
if v.dim() != 3:
print("velocity's dim must be 3 for batch operation")
exit(-1)
on_gpu = v.is_cuda
if not on_gpu and torch.cuda.is_available():
v = v.cuda()
padding_size = window_size - 1
batch_size = v.shape[0]
pad = v[:, 0, :].reshape(batch_size, 1, 3).expand(batch_size, padding_size, 3)
v_normed = torch.cat([pad, v], dim=1)
for i in range(window_size-1, 0, -1):
v_normed[:, i-1:i-1+N, :] += v
v_normed = v_normed[:, :N, :] / float(window_size)
v_normed = v - v_normed
if not on_gpu:
v_normed = v_normed.cpu()
return v_normed | 69f3c8cd6a5628d09a7fd78f3b5b779611a68411 | 3,459 |
def viz_property(statement, properties):
"""Create properties for graphviz element"""
if not properties:
return statement + ";";
return statement + "[{}];".format(" ".join(properties)) | 518d4a662830737359a8b3cb9cec651394823785 | 3,461 |
import platform
import subprocess
import re
def detect_windows_needs_driver(sd, print_reason=False):
"""detect if Windows user needs to install driver for a supported device"""
need_to_install_driver = False
if sd:
system = platform.system()
#print(f'in detect_windows_needs_driver system:{system}')
if system == "Windows":
# if windows, see if we can find a DeviceId with the vendor id
# Get-PnpDevice | Where-Object{ ($_.DeviceId -like '*10C4*')} | Format-List
command = 'powershell.exe "[Console]::OutputEncoding = [Text.UTF8Encoding]::UTF8; Get-PnpDevice | Where-Object{ ($_.DeviceId -like '
command += f"'*{sd.usb_vendor_id_in_hex.upper()}*'"
command += ')} | Format-List"'
#print(f'command:{command}')
_, sp_output = subprocess.getstatusoutput(command)
#print(f'sp_output:{sp_output}')
search = f'CM_PROB_FAILED_INSTALL'
#print(f'search:"{search}"')
if re.search(search, sp_output, re.MULTILINE):
need_to_install_driver = True
# if the want to see the reason
if print_reason:
print(sp_output)
return need_to_install_driver | 52e681152442b27944396bf35d6a7c80a39b8639 | 3,462 |
import csv
def read_loss_file(path):
"""Read the given loss csv file and process its data into lists that can be
plotted by matplotlib.
Args:
path (string): The path to the file to be read.
Returns: A list of lists, one list for each subnetwork containing the loss
values over time.
"""
with open(path, 'r') as csvfile:
reader = csv.reader(csvfile)
data = []
for row in reader:
# Ignore the epoch numbers
if len(data) == 0:
data = [[] for _ in row[1:]]
for i in range(1, len(row)):
data[i-1].append(float(row[i]))
return data | 8e861f0bf46db5085ea2f30a7e70a4bdfa0b9697 | 3,463 |
import os
def xml_files_list(path):
"""
Return the XML files found in `path`
"""
return (f for f in os.listdir(path) if f.endswith(".xml")) | 27cc2769e34f55263c60ba07a92e181bfec641ab | 3,465 |
import torch
def make_observation_mapper(claims):
"""Make a dictionary of observation.
Parameters
----------
claims: pd.DataFrame
Returns
-------
observation_mapper: dict
an dictionary that map rv to their observed value
"""
observation_mapper = dict()
for c in claims.index:
s = claims.iloc[c]['source_id']
observation_mapper[f'b_{s}_{c}'] = torch.tensor(
claims.iloc[c]['value'])
return observation_mapper | 43052bd9ce5e1121f3ed144ec48acf20ad117313 | 3,467 |
def simplify(n):
"""Remove decimal places."""
return int(round(n)) | 9856c8f5c0448634956d1d05e44027da2f4ebe6a | 3,468 |
import os
def get_ids(viva_path, dataset):
"""Get image identifiers for corresponding list of dataset identifies.
Parameters
----------
viva_path : str
Path to VIVA directory.
datasets : list of str tuples
List of dataset identifiers in the form of (year, dataset) pairs.
Returns
-------
ids : list of str
List of all image identifiers for given datasets.
"""
dataset_path = os.path.join(viva_path, dataset, 'pos')
ids = [dir.replace('.png', '') for dir in os.listdir(dataset_path)]
return ids | 526c094323af2be7611c3bf9261c703106442b24 | 3,469 |
import math
def VSphere(R):
"""
Volume of a sphere or radius R.
"""
return 4. * math.pi * R * R * R / 3. | 9e99d19683d9e86c2db79189809d24badccc197b | 3,470 |
import os
def generate_navbar(structure, pathprefix):
"""
Returns 2D list containing the nested navigational structure of the website
"""
navbar = []
for section in structure['sections']:
navbar_section = []
section_data = structure['sections'][section]
section_url = os.path.join('/', pathprefix, section + '/')
section_title = section_data['title']
if 'navtitle' in section_data:
section_title = section_data['navtitle']
section_hassub = False
if 'pages' in section_data:
section_hassub = True
for page in section_data['pages']:
url = os.path.join('/', pathprefix, section, page)
title = structure['sections'][section]['pages'][page]['title']
if 'navtitle' in structure['sections'][section]['pages'][page]:
title = structure['sections'][section]['pages'][page]['navtitle']
if title:
# Only add a page to the navigation if it has a title, otherwise it's hidden
navbar_section.append((url, page, title))
if section_title:
navbar.append((section_url, section, section_title, section_hassub, navbar_section))
return navbar | daed8a1a74887406700fc2ca380e22165fa300f6 | 3,471 |
import os
def extract_group_ids(caps_directory):
"""Extract list of group IDs (e.g. ['group-AD', 'group-HC']) based on `caps_directory`/groups folder."""
try:
group_ids = os.listdir(os.path.join(caps_directory, "groups"))
except FileNotFoundError:
group_ids = [""]
return group_ids | 908ac1c3c06028f526b1d474618fca05d76f8792 | 3,472 |
import re
def decode_Tex_accents(in_str):
"""Converts a string containing LaTex accents (i.e. "{\\`{O}}") to ASCII
(i.e. "O"). Useful for correcting author names when bib entries were
queried from web via doi
:param in_str: input str to decode
:type in_str: str
:return: corrected string
:rtype: str
"""
# replaces latex accents with ascii letter (no accent)
pat = "\{\\\\'\{(\w)\}\}"
out = in_str
for x in re.finditer(pat, in_str):
out = out.replace(x.group(), x.groups()[0])
# replace latex {\textsinglequote} with underscore
out = out.replace('{\\textquotesingle}', "_")
# replace actual single quotes with underscore for bibtex compatibility
out = out.replace("'", '_')
return out | 2a4bd71b53cdab047a1ddd1e0e6fd6e9c81b0e0a | 3,473 |
def getScale(im, scale, max_scale=None):
"""
获得图片的放缩比例
:param im:
:param scale:
:param max_scale:
:return:
"""
f = float(scale) / min(im.shape[0], im.shape[1])
if max_scale != None and f * max(im.shape[0], im.shape[1]) > max_scale:
f = float(max_scale) / max(im.shape[0], im.shape[1])
return f | 52ae195714c1d39bccec553797bf6dbf2c6c2795 | 3,474 |
import os
def getFileName(in_path, with_extension=False):
"""Return the file name with the file extension appended.
Args:
in_path (str): the file path to extract the filename from.
with_extension=False (Bool): flag denoting whether to return
the filename with or without the extension.
Returns:
Str - file name, with or without the extension appended or a
blank string if there is no file name in the path.
"""
filename = os.path.basename(in_path)
if os.path.sep in in_path[-2:] or (with_extension and not '.' in filename):
return ''
if with_extension:
return filename
else:
filename = os.path.splitext(filename)[0]
return filename | 56972e397a2b5961656175962ab799bb95761e7c | 3,475 |
def answer_question_interactively(question):
"""Returns True or False for t yes/no question to the user"""
while True:
answer = input(question + '? [Y or N]: ')
if answer.lower() == 'y':
return True
elif answer.lower() == 'n':
return False | 52a123cc2237441de3b0243da268e53b7cc0d807 | 3,476 |
def other_players(me, r):
"""Return a list of all players but me, in turn order starting after me"""
return list(range(me+1, r.nPlayers)) + list(range(0, me)) | 5c2d2b03bfb3b99eb4c347319ccaaa3fc495b6c4 | 3,477 |
def to_bytes(obj, encoding='utf-8', errors='strict'):
"""Makes sure that a string is a byte string.
Args:
obj: An object to make sure is a byte string.
encoding: The encoding to use to transform from a text string to
a byte string. Defaults to using 'utf-8'.
errors: The error handler to use if the text string is not
encodable using the specified encoding. Any valid codecs error
handler may be specified.
Returns: Typically this returns a byte string.
"""
if isinstance(obj, bytes):
return obj
return bytes(obj, encoding=encoding, errors=errors) | 4f8a0dcfdcfd3e2a77b5cbeedea4cb2a11acd4c1 | 3,480 |
def options():
"""Stub version of the parsed command line options."""
class StubOptions(object):
profile = None
return StubOptions() | dea85d2956eb6cbf97f870a74b122896915c8c19 | 3,481 |
def midnight(date):
"""Returns a copy of a date with the hour, minute, second, and
millisecond fields set to zero.
Args:
date (Date): The starting date.
Returns:
Date: A new date, set to midnight of the day provided.
"""
return date.replace(hour=0, minute=0, second=0, microsecond=0) | b92086dd9d99a4cea6657d37f40e68696ad41f7c | 3,483 |
from typing import Callable
from typing import Any
from typing import Sequence
def foldr(fun: Callable[[Any, Any], Any], acc: Any, seq: Sequence[Any]) -> Any:
"""Implementation of foldr in Python3.
This is an implementation of the right-handed
fold function from functional programming.
If the list is empty, we return the accumulator
value. Otherwise, we recurse by applying the
function which was passed to the foldr to
the head of the iterable collection
and the foldr called with fun, acc, and
the tail of the iterable collection.
Below are the implementations of the len
and sum functions using foldr to
demonstrate how foldr function works.
>>> foldr((lambda _, y: y + 1), 0, [0, 1, 2, 3, 4])
5
>>> foldr((lambda x, y: x + y), 0, [0, 1, 2, 3, 4])
10
foldr takes the second argument and the
last item of the list and applies the function,
then it takes the penultimate item from the end
and the result, and so on.
"""
return acc if not seq else fun(seq[0], foldr(fun, acc, seq[1:])) | 5648d8ce8a2807270163ebcddad3f523f527986e | 3,484 |
def filter_chants_without_volpiano(chants, logger=None):
"""Exclude all chants with an empty volpiano field"""
has_volpiano = chants.volpiano.isnull() == False
return chants[has_volpiano] | 3f03bbf3f247afd3a115442e8121a773aa90fb56 | 3,485 |
import pathlib
import os
def get_builtin_templates_path() -> pathlib.Path:
"""Return the pathlib.Path to the package's builtin templates
:return: template pathlib.Path
"""
return pathlib.Path(
os.path.dirname(os.path.realpath(__file__))
) / 'templates' | 4b6bfeb826aa2badb000267e12a9bb6cf19f6a28 | 3,486 |
def extract_row_loaded():
"""extract_row as it should appear in memory"""
result = {}
result['classification_id'] = '91178981'
result['user_name'] = 'MikeWalmsley'
result['user_id'] = '290475'
result['user_ip'] = '2c61707e96c97a759840'
result['workflow_id'] = '6122'
result['workflow_name'] = 'DECaLS DR5'
result['workflow_version'] = '28.30'
result['created_at'] = '2018-02-20 10:44:42 UTC'
result['gold_standard'] = ''
result['expert'] = ''
result['metadata'] = {
'session': 'e69d40c94873e2e4e2868226d5567e0e997bf58e8800eef4def679ff3e69f97f',
'viewport': {
'width': 1081,
'height': 1049
},
'started_at':'2018-02-20T10:41:13.381Z',
'user_agent': 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10.12; rv:58.0) Gecko/20100101 Firefox/58.0',
'utc_offset': '0',
'finished_at': '2018-02-20T10:44:42.480Z',
'live_project': True,
'user_language': 'en',
'user_group_ids':[],
'subject_dimensions': [{
'clientWidth': 424,
'clientHeight': 424,
'naturalWidth': 424,
'naturalHeight': 424
}]}
result['annotations'] = [
{
'task': 'T0',
'task_label': 'Is the galaxy simply smooth and rounded, with no sign of a disk?',
'value':'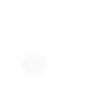 Features or Disk'
},
{
'task': 'T2',
'task_label': 'Could this be a disk viewed edge-on?',
'value':'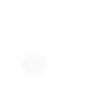 No'
},
{
'task': 'T4',
'task_label': 'Is there a bar feature through the centre of the galaxy?',
'value': 'No Bar'
},
{
'task': 'T5',
'task_label': 'Is there any sign of a spiral arm pattern?',
'value': 'Yes'
},
{
'task': 'T6',
'task_label': 'How tightly wound do the spiral arms appear?',
'value': '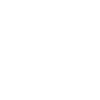 Tight'
},
{
'task': 'T7',
'task_label':'How many spiral arms are there?',
'value':'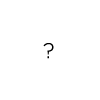 Cant tell'
},
{
'task':'T8',
'task_label':'How prominent is the central bulge, compared with the rest of the galaxy?',
'value':'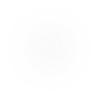 No bulge'
},
{
'task':'T11',
'task_label':'Is the galaxy currently merging, or is there any sign of tidal debris?',
'value':'Neither'
},
{
'task':'T10',
'task_label':'Do you see any of these rare features in the image?',
'value':[]
}
]
result['subject_data'] = {
'15715879': {
'retired': None,
'ra': 319.11521779916546,
'dec': -0.826509379829966,
'mag.g': 13.674222230911255,
'mag.i': 12.560198307037354,
'mag.r': 12.938228249549866,
'mag.u': 15.10558009147644,
'mag.z':12.32387661933899,
'nsa_id':189862.0,
'redshift':0.019291512668132782,
'mag.abs_r':-20.916738510131836,
'mag.faruv':16.92647397518158,
'petroflux':5388.59814453125,
'petroth50':13.936717987060547,
'mag.nearuv':16.298240423202515,
'petrotheta':28.682878494262695,
'absolute_size':11.334824080956198
}
}
result['subject_ids'] = '15715879'
return result | c5fd87149ab7dff6e9980a898013053ce309c259 | 3,487 |
def bitbucketBaseIssue():
"""
BitbucketIssueのベースとなるデータを生成して返します。
"""
return {
'assignee': None,
'component': None,
'content': 'issue_summary',
'content_updated_on': '4000-01-01T00:00:00Z',
'created_on': '3000-01-01T00:00:00Z',
'edited_on': '4000-01-01T00:00:00Z',
'id': 1,
'kind': 'task',
'milestone': None,
'priority': 'major',
'reporter': {
'display_name': 'user_name',
'account_id': 'user_name'
},
'status': 'open',
'title': 'issue_title',
'updated_on': '4000-01-01T00:00:00Z',
'version': None,
'watchers': [],
'voters': []
} | b0a81a9442377967b0838ffe49468dd21631b1c0 | 3,488 |
from typing import List
from typing import Dict
def get_events() -> List[Dict]:
"""Represents set of sales events"""
return [{
"Txid": 1,
"productname": "Product 2",
"qty": 2,
"sales": 489.5
}, {
"Txid": 2,
"productname": "Product 3 XL",
"qty": 2,
"sales": 411.8
}, {
"Txid": 3,
"productname": "Product 4",
"qty": 2,
"sales": 56.15
}, {
"Txid": 4,
"productname": "Product 4 XL",
"qty": 5,
"sales": 197.7
}, {
"Txid": 5,
"productname": "Product 3",
"qty": 7,
"sales": 222.3
}] | 22361fc66926a9adb5b10f4ad9ba04767a7d7a25 | 3,490 |
def sec2hms(sec):
"""
Convert seconds to hours, minutes and seconds.
"""
hours = int(sec/3600)
minutes = int((sec -3600*hours)/60)
seconds = int(sec -3600*hours -60*minutes)
return hours,minutes,seconds | efea3a641c5f13313adb571c201cc25d2895757e | 3,492 |
def spectre_avatar(avatar, size="sm", **kwargs):
"""
render avatar
:param avatar:
:param size:
:param kwargs:
:return: HTML
"""
avatar_kwargs = kwargs.copy()
avatar_kwargs['avatar_url'] = avatar
avatar_kwargs['size'] = size
if "background" not in avatar_kwargs.keys():
avatar_kwargs['background'] = "#5755d9"
return avatar_kwargs | d573336c13f63cb647d40241d2c37f78fd4cc292 | 3,494 |
def do_json_counts(df, target_name):
""" count of records where name=target_name in a dataframe with column 'name' """
return df.filter(df.name == target_name).count() | c4b0cb52f28372a7d53a92984b3212c66c1556ab | 3,495 |
def union(a, b):
"""Find union of two lists, sequences, etc.
Returns a list that includes repetitions if they occur in the input lists.
"""
return list(a) + list(b) | bf154b6222122aa61cf590022475dcd38eed6df4 | 3,496 |
def user_prompt(msg: str, sound: bool = False, timeout: int = -1):
"""Open user prompt."""
return f'B;UserPrompt("{msg}",{sound:d},{timeout});'.encode() | 25e2941c212c487f9a319159a3eab66d3ff38050 | 3,498 |
def count_subset_sum_recur(arr, total, n):
"""Count subsets given sum by recusrion.
Time complexity: O(2^n), where n is length of array.
Space complexity: O(1).
"""
if total < 0:
return 0
if total == 0:
return 1
if n < 0:
return 0
if total < arr[n]:
return count_subset_sum_recur(arr, total, n - 1)
else:
n_subsets_in = count_subset_sum_recur(arr, total - arr[n], n - 1)
n_subsets_out = count_subset_sum_recur(arr, total, n - 1)
return n_subsets_in + n_subsets_out | 981b83014e75122dea814ace5b34b18f9803c3ad | 3,499 |
import os
def get_test_files(dirname):
"""
Gets a list of files in directory specified by name.
"""
if not os.path.isdir(dirname):
return []
path = dirname + "/{}"
return list(map(path.format, sorted(os.listdir(dirname)))) | 8c9f4e47837534a0a826881cfe037f0042df9010 | 3,500 |
def get_indexes_from_list(lst, find, exact=True):
"""
Helper function that search for element in a list and
returns a list of indexes for element match
E.g.
get_indexes_from_list([1,2,3,1,5,1], 1) returns [0,3,5]
get_indexes_from_list(['apple','banana','orange','lemon'], 'orange') -> returns [2]
get_indexes_from_list(['apple','banana','lemon',['orange', 'peach']], 'orange') -> returns []
get_indexes_from_list(['apple','banana','lemon',['orange', 'peach']], ['orange'], False) -> returns [3]
Parameters
----------
lst: list
The list to look in
find: any
the element to find, can be a list
exact: bool
If False then index are returned if find in lst-item otherwise
only if find = lst-item
Returns
-------
list of int
"""
if exact == True:
return [index for index, value in enumerate(lst) if value == find]
else:
if isinstance(find,list):
return [index for index, value in enumerate(lst) if set(find).intersection(set(value))]
else:
return [index for index, value in enumerate(lst) if find in value] | 416d94de975603a60bf41974b8564cd868e503c0 | 3,502 |
def looks_like_PEM(text):
"""
Guess whether text looks like a PEM encoding.
"""
i = text.find("-----BEGIN ")
return i >= 0 and text.find("\n-----END ", i) > i | ff310d6ffcf6d4ebd63331fdae66774009ef2b39 | 3,503 |
from typing import Counter
def find_duplicates(list_to_check):
"""
This finds duplicates in a list of values of any type and then returns the values that are duplicates. Given Counter
only works with hashable types, ie it can't work with lists, create a tuple of the lists and then count if the
list_to_check contains un-hashable items
:param list_to_check: A list of values with potential duplicates within it
:type list_to_check: list
:return:The values that where duplicates
:rtype: list
"""
try:
counted_list = Counter(list_to_check)
except TypeError:
counted_list = Counter([tuple(x) for x in list_to_check])
return [key for key in counted_list if counted_list[key] > 1] | 1d608a70e7fb9be2001c73b72d3c1b62047539b5 | 3,504 |
from typing import Any
def none_to_default(field: Any, default: Any) -> Any:
"""Convert None values into default values.
:param field: the original value that may be None.
:param default: the new, default, value.
:return: field; the new value if field is None, the old value
otherwise.
:rtype: any
"""
return default if field is None else field | 894d71c2cc89b02dc14fd7ddcd3a949bdc336692 | 3,505 |
def stringify_dict_key(_dict):
"""
保证_dict中所有key为str类型
:param _dict:
:return:
"""
for key, value in _dict.copy().items():
if isinstance(value, dict):
value = stringify_dict_key(value)
if not isinstance(key, str):
del _dict[key]
_dict[str(key)] = value
return _dict | e83df720772aa4122d9a435da20279d4a800e074 | 3,506 |
def GetFlippedPoints3(paths, array):
"""same as first version, but doesnt flip locations: just sets to -1
used for random walks with self intersections - err type 6"""
# this may not work for double ups?
for i in paths:
for j in i: # for the rest of the steps...
array[j[0]][j[1]][j[2]] = -1 # flip initial position
return(array) | ad0bc7a03e293beb2542ee555a341bdfc8706408 | 3,507 |
def gen_input_code(question, id):
"""
Returns the html code for rendering the appropriate input
field for the given question.
Each question is identified by name=id
"""
qtype = question['type']
if qtype == 'text':
return """<input type="text" class="ui text" name="{0}"
placeholder="your answer..." />""".format(id)
elif qtype == 'code':
return '<textarea class="ui text" name="{0}"></textarea>'.format(id)
else:
button_template = '<input type="radio" name="{0}" value="{1}"> {1}<br>'
code = ''
for choice in question['choices']:
code = code + button_template.format(id, choice)
return code | b76bea45c0ce847d664a38694732ef0b75c2a53c | 3,508 |
def list_inventory (inventory):
"""
:param inventory: dict - an inventory dictionary.
:return: list of tuples - list of key, value pairs from the inventory dictionary.
"""
result = []
for element, quantity in inventory.items():
if quantity > 0:
result.append ((element, quantity))
return result | 264f8cde11879be8ace938c777f546974383122c | 3,509 |
def measure_approximate_cost(structure):
""" Various bits estimate the size of the structures they return. This makes that consistent. """
if isinstance(structure, (list, tuple)): return 1 + sum(map(measure_approximate_cost, structure))
elif isinstance(structure, dict): return len(structure) + sum(map(measure_approximate_cost, structure.values()))
elif isinstance(structure, int) or structure is None: return 1
else: assert False, type(structure) | 8adbd962e789be6549745fbb71c71918d3cc8d0c | 3,510 |
import hashlib
def get_fingerprint(file_path: str) -> str:
"""
Calculate a fingerprint for a given file.
:param file_path: path to the file that should be fingerprinted
:return: the file fingerprint, or an empty string
"""
try:
block_size = 65536
hash_method = hashlib.md5()
with open(file_path, 'rb') as input_file:
buf = input_file.read(block_size)
while buf:
hash_method.update(buf)
buf = input_file.read(block_size)
return hash_method.hexdigest()
except Exception:
# if the file cannot be hashed for any reason, return an empty fingerprint
return '' | b0ee4d592b890194241aaafb43ccba927d13662a | 3,511 |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.